Numerical Examples
This page illustrates how FsRandom is applicable to scientific computations. For statistics, the Statistics module is useful:
1:
|
|
Estimating pi, the ratio of a circle's circumference to its diameter
Suppose there is a circle of radius 1 is inside a square with side length 2. The area of the circle is \(\pi\) and the area of the square is 4. If you put \(N\) random points on the square, roughly \(\displaystyle\frac{\pi}{4}N\) points are inside the circle. In other words, if you find \(M\) points out of \(N\) are inside the circle, \(\displaystyle\frac{M}{N}\) approximates \(\displaystyle\frac{\pi}{4}\).
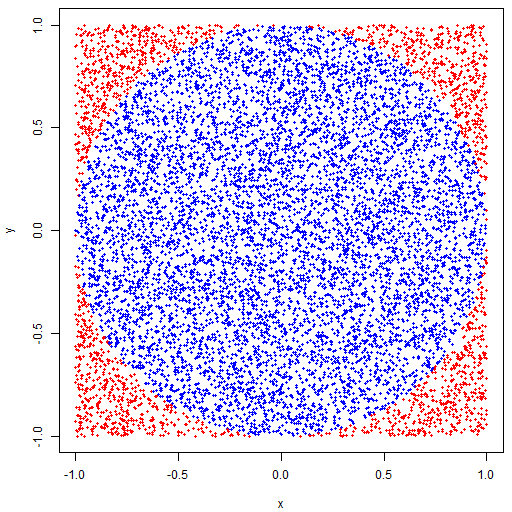
Random points can be described simply and easily as follows:
1: 2: 3: 4: 5: |
|
To give each point weight 4 if the point is inside the circle and 0 otherwise adjusts the average of total score to become \(\pi\).
1: 2: |
|
The average of the random scores approximates \(\pi\). To generate 1,000,000 scores and to compute the approximation:
1: 2: 3: 4: |
|
|
Generating bivariate normal random numbers using Gibbs sampler
To sample from bivariate normal distribution \(\displaystyle N_{2}\left( \left[\begin{array}{c} \mu_{X} \\ \mu_{Y} \end{array}\right], \left[\begin{array}{cc} \sigma_{X}^{2} & \sigma_{XY} \\ \sigma_{XY} & \sigma_{Y}^{2} \end{array}\right] \right) \), you will construct a Gibbs sampler. Let \(f_{2}(x, y)\) be the density function of \(N_{2}\), and let \(x'\) and \(y'\) be \(x-\mu_{X}\) and \(y-\mu_{Y}\) respectively. Then, \[\begin{eqnarray} f\_{2}(x, y) & \propto & \exp\left( -\frac{1}{2}\left[\begin{array}{c} x' \\\\ y' \end{array}\right]^{T} \left[\begin{array}{cc} \sigma\_{X}^{2} & \sigma\_{XY} \\\\ \sigma\_{XY} & \sigma\_{Y}^{2} \end{array}\right]^{-1} \left[\begin{array}{c} x' \\\\ y' \end{array}\right] \right) \\\\ & \propto & \exp\left( -\frac{\left(x'-\sigma\_{XY}y'/\sigma\_{Y}^{2}\right)^{2}}{2\left(\sigma\_{X}^{2}-\sigma\_{XY}^{2}/\sigma\_{Y}^{2}\right)} \right) \end{eqnarray}\] This means the conditional probability of \(x\) given \(y\) is distributed normally, and its mean is \(\displaystyle \mu_{X}+\frac{\sigma_{XY}}{\sigma_{Y}^{2}}\left(y-\mu_{Y}\right)\) and its variance is \(\displaystyle \sigma_{X}^{2}-\frac{\sigma_{XY}^{2}}{\sigma_{Y}^{2}}\). Therefore, the Gibbs sampler for bivariate normal distribution consists of iterating as the following:
- Draw \(\displaystyle x_{t+1}\sim N\left(\mu_{X}+\frac{\sigma_{XY}}{\sigma_{Y}^{2}}(y_{t}-\mu_{Y}), \sigma_{X}^{2}-\frac{\sigma_{XY}^{2}}{\sigma_{Y}^{2}}\right)\)
- Draw \(\displaystyle y_{t+1}\sim N\left(\mu_{Y}+\frac{\sigma_{XY}}{\sigma_{X}^{2}}(x_{t+1}-\mu_{Y}), \sigma_{Y}^{2}-\frac{\sigma_{XY}^{2}}{\sigma_{X}^{2}}\right)\)
And it can be naturally translated into F# code as the following.
1: 2: 3: 4: 5: 6: 7: 8: 9: |
|
Note that the generating bivariate normal random number sequence is autocorrelated.
Hamiltonian Monte Carlo
Gibbs sampler sometimes produces strongly autocorrelated traces. Hamiltonian Monte Carlo (also known as hybrid Monte Carlo) could be efficient in such situations. Hamiltonian Monte Carlo algorithm is available if you know about the density of the taget distribution without normalizing constant.
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: 12: 13: 14: 15: 16: 17: 18: 19: 20: 21: 22: 23: 24: 25: 26: 27: 28: 29: 30: 31: 32: |
|
Approximate Bayesian Computation
Approximate Bayesian computation is known as a likelihood-free method of parameter estimation. This section follows the example in the Wikipedia article.
The initial state of the system is not described whether it is determined or inferred. Here it is assumed as 'A' for convenience. Then, the model is described as follows:
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: 12: 13: 14: 15: |
|
Step 1: the observed data is AAAABAABBAAAAAABAAAA
.
1:
|
|
Step 2: the prior of theta is a uniform [0, 1] and gamma is known.
1: 2: |
|
Step 3: the summary statistic is the frequency of switches between two states (A and B). Note that the summary statistic is bad to estimate theta (see the article).
1: 2: 3: 4: |
|
Step 4: the distance between the observed and simulated is the difference between the summary statistics.
1:
|
|
Step 5: do the simulation with tolerance epsilon.
1: 2: 3: 4: 5: 6: 7: 8: |
|
Full name: Numerical-examples.state
Full name: FsRandom.RandomNumberGenerator.createState
Full name: FsRandom.RandomNumberGenerator.xorshift
from FsRandom
Full name: Numerical-examples.randomPointGenerator
Full name: FsRandom.RandomNumberGenerator.random
Full name: FsRandom.Statistics.uniform
Full name: Numerical-examples.weight
Full name: Numerical-examples.randomScoreGenerator
from FsRandom
Full name: FsRandom.Random.map
module Seq
from FsRandom.StatisticsModule
--------------------
module Seq
from FsRandom
--------------------
module Seq
from Microsoft.FSharp.Collections
Full name: FsRandom.Seq.ofRandom
Full name: Microsoft.FSharp.Collections.Seq.take
Full name: Microsoft.FSharp.Collections.Seq.average
Full name: Microsoft.FSharp.Core.ExtraTopLevelOperators.printf
Full name: Numerical-examples.gibbsBinormal
Full name: Microsoft.FSharp.Core.Operators.sqrt
Full name: FsRandom.Statistics.normal
Full name: Numerical-examples.binormal
Full name: FsRandom.StatisticsModule.Seq.markovChain
Full name: Numerical-examples.updateWith
val float : value:'T -> float (requires member op_Explicit)
Full name: Microsoft.FSharp.Core.Operators.float
--------------------
type float = System.Double
Full name: Microsoft.FSharp.Core.float
--------------------
type float<'Measure> = float
Full name: Microsoft.FSharp.Core.float<_>
module Array
from FsRandom
--------------------
module Array
from Microsoft.FSharp.Collections
Full name: Microsoft.FSharp.Collections.Array.length
Full name: Numerical-examples.hmc
Hamiltonian Monte Carlo
Leapfrog integration
Hamiltonian
Full name: Microsoft.FSharp.Collections.Array.fold
resampling of particles
Full name: FsRandom.Array.randomCreate
from FsRandom.StatisticsModule
Full name: FsRandom.StatisticsModule.Standard.normal
Full name: Microsoft.FSharp.Collections.Array.copy
Full name: FsRandom.RandomNumberGenerator.( [0, 1) )
Full name: Microsoft.FSharp.Core.Operators.exp
| A
| B
Full name: Numerical-examples.HiddenSystem
Full name: Numerical-examples.switch
Full name: Numerical-examples.observe
Full name: Numerical-examples.model
val ref : value:'T -> 'T ref
Full name: Microsoft.FSharp.Core.Operators.ref
--------------------
type 'T ref = Ref<'T>
Full name: Microsoft.FSharp.Core.ref<_>
type StringBuilder =
new : unit -> StringBuilder + 5 overloads
member Append : value:string -> StringBuilder + 19 overloads
member AppendFormat : format:string * arg0:obj -> StringBuilder + 7 overloads
member AppendLine : unit -> StringBuilder + 1 overload
member Capacity : int with get, set
member Chars : int -> char with get, set
member Clear : unit -> StringBuilder
member CopyTo : sourceIndex:int * destination:char[] * destinationIndex:int * count:int -> unit
member EnsureCapacity : capacity:int -> int
member Equals : sb:StringBuilder -> bool
...
Full name: System.Text.StringBuilder
--------------------
System.Text.StringBuilder() : unit
System.Text.StringBuilder(capacity: int) : unit
System.Text.StringBuilder(value: string) : unit
System.Text.StringBuilder(value: string, capacity: int) : unit
System.Text.StringBuilder(capacity: int, maxCapacity: int) : unit
System.Text.StringBuilder(value: string, startIndex: int, length: int, capacity: int) : unit
from FsRandom
Full name: FsRandom.Utility.flipCoin
(+0 other overloads)
System.Text.StringBuilder.Append(value: obj) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: uint64) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: uint32) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: uint16) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: decimal) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: float) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: float32) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: int64) : System.Text.StringBuilder
(+0 other overloads)
System.Text.StringBuilder.Append(value: int) : System.Text.StringBuilder
(+0 other overloads)
Full name: Microsoft.FSharp.Core.Operators.ignore
System.Text.StringBuilder.ToString(startIndex: int, length: int) : string
Full name: Numerical-examples.observed
Full name: Numerical-examples.prior
Full name: Numerical-examples.gamma
Full name: Numerical-examples.w
val string : value:'T -> string
Full name: Microsoft.FSharp.Core.Operators.string
--------------------
type string = System.String
Full name: Microsoft.FSharp.Core.string
Full name: Microsoft.FSharp.Collections.Seq.windowed
Full name: Microsoft.FSharp.Collections.Seq.filter
Full name: Microsoft.FSharp.Collections.Seq.length
Full name: Numerical-examples.rho
Full name: Microsoft.FSharp.Core.Operators.abs
| Accept of float
| Reject
Full name: Numerical-examples.SimulationResult
Full name: Numerical-examples.simulate
module String
from FsRandom
--------------------
module String
from Microsoft.FSharp.Core
Full name: Microsoft.FSharp.Core.String.length